On this page |
The public API is available for C++, Blueprints, and Python.
The public API supports the following:
-
instiantate HDas as actors in a world
-
setting parameters and inputs
-
cooking HDAs
-
inspect, interate, and bake outputs.
Houdini Public API Instance ¶
The class UHoudiniPublicAPI
provides some functions to control the session and instantiate Houdini asset actors.
Command |
Function |
---|---|
|
Returns true if a valid Houdini Engine session is running/connected |
|
Start a new Houdini Engine Session if there is no current session |
|
Stops the current Houdini Engine session |
|
Stops, then creates a new Houdini Engine session |
|
Instantiates an HDA in the specified world/level. Returns a wrapper for instantiated asset. See Instantiating HDAs |
|
Returns true if asset cooking is paused |
|
Pause asset cooking (if not already paused) |
|
Resume asset cooking (if it was paused) |
|
Create a new empty API input object. The user must populate it and then set it as an input on an asset wrapper. See Inputs. See Inputs |
Instantiating HDAs ¶
The InstantiateAsset
function returns an API wrapper class (UHoudiniPublicAPIAssetWrapper
) to interact with the instantiated HDA
Warning
The Public API does not manage the lifecycle of the UHoudiniPublicAPIAssetWrapper
after creation with InstantiateAsset
. Use a UProperty
or Blueprint variable to prevent unexpected garbage collection of the wrapper.
Command |
Function |
---|---|
|
The HDA uasset to instantiate |
|
The Transform to instantiate the HDA actor |
|
A world context object for identifying the world to spawn, if |
|
If not |
|
If true (the default) the HDA cooks automatically after instantiation and after parameter, transform, and input changes |
|
If true, the HDA output is automatically baked after a cook. Default is false |
|
The directory to bake to if the bake path is not set in attributes on the HDA output |
|
The bake target (to actor vs blueprint) |
|
If true, HDA temporary outputs are removed after a bake. Default is false |
|
Recenter the baked actors to their bounding box center. Default is false |
|
If true, on every bake replace the previous bake’s output (assets and actors) with the new bake’s output. Default is false |
Public API Asset Wrapper ¶
The API wraps instantiated HDAs in instances of UHoudiniPublicAPIAssetWrapper
. This class allows you to manipulate the instantiated HDA by setting parameters, inputs, cooking, baking, and accessing outputs.
Creating A Wrapper For An Existing HDA Actor ¶
The Instantiating HDAs section discusses the InstantiateAsset
function that instantiates a new HDA and returns a UHoudiniPublicAPIAssetWrapper
, but you can also create a wrapper for an existing HDA actor/ component. The CreateWrapper
static function of UHoudiniPublicAPIAssetWrapper
(also available in editor utility blueprints as Create Wrapper
) takes an existing HDA actor or component as an argument and creates and returns a UHoudiniPublicAPIAssetWrapper
that wraps the specified HDA actor / component.
Alternatively, you can also use the CreateEmptyWrapper
function to create a UHoudiniPublicAPIAssetWrapper
that does not initially wrap any HDA actor/component. You can then use the WrapHoudiniAssetObject
function of UHoudiniPublicAPIAssetWrapper
to wrap an existing HDA actor/component with the wrapper.
Warning
The Public API does not manage the lifecycle of the UHoudiniPublicAPIAssetWrapper
after creation with CreateEmptyWrapper
or CreateWrapper
. Use a UProperty
or Blueprint variable to prevent unexpected garbage collection of the wrapper.
Delegates ¶
In many cases, plugin interacts with the Houdini Engine asynchrously. This means after an operation completes, you need to bind to a delegate to recieve a callback.
An example is if you want to change a parameter, the node cooks then fetches the output. You need to bind to the proper delegate to receive a callback once the process completes.
Command |
Function |
---|---|
|
Broadcast before an HDA is instantiated. The HDA’s default parameters are available and can be set |
|
Broadcast after the asset was successfully instantiated. Recommended to set/configure inputs before the first cook |
|
Broadcast after a cook completes. Output objects/assets not yet created or updated |
|
Broadcast at the end of the pre-processing phase (after a cook). Output objects/assets not yet created or updated |
|
Broadcast after output processing in Unreal. Output objects/assets not yet created or updated |
|
Broadcast after baking the asset (not called for individual outputs baked to the content browser) |
|
Broadcast after a cook of a TOP network completes. Work item results not necessarily loaded |
|
Broadcast after baking PDG outputs (not called for individual outputs baked to the content browser) |
|
Broadcast after refining all proxy meshes for this wrapped asset |
Note
In blueprints the public API is only available in editor utility graphs: any blueprint event or function bound to a delegate must have Call In Editor enabled otherwise the event/function will not execute when broadcasting the delegate.
Parameters ¶
The following functions can set and get parameter values of a named parameter tuple. The parameter tuple name is a required argument and the index of the parameter in the tuple is optional (default to 0).
Command |
Function |
---|---|
|
Set the value of a float parameter |
|
Get the value of a float parameter |
|
Set the value of a float parameter |
|
Get the value of a float parameter |
|
Set the value of a integer parameter |
|
Get the value of a integer parameter |
|
Set the value of a boolean/toggle parameter |
|
Get the value of a boolean/toggle parameter |
|
Set the value of a string parameter |
|
Get the value of a string parameter |
|
Set the value of string parameter from a UObject as an asset reference |
|
Get the UObject instance referenced by a string parameter |
|
Executes the callback script of a button parameter |
|
Gets the values of all parameter tuples as an array of structs |
|
Sets the values of the parameter tuples in the given array of structs |
The following functions help simplify working with ramp parameters:
Command |
Function |
---|---|
|
Set the number of points of ramp parameter |
|
Get the number of points of ramp parameter |
|
Set the point position, value and interpolation of a float ramp point by index |
|
Get the point position, value and interpolation of a float ramp point by index |
|
Set all of the points of a float ramp parameter from an array |
|
Get all of the points of a float ramp parameter as an array. |
|
Set the point position, value and interpolation of a color ramp point by index |
|
Get the point position, value and interpolation of a color ramp point by index |
|
Set all of the points of a color ramp parameter from an array |
|
Get all of the points of a color ramp parameter as an array. |
Inputs ¶
Inputs types are supported in API wrapper classes.
To set an input on a wrapped instantiated HDA, instantiate the appropriate input class and then set the input objects and settings. You can instantiate inputs using the UHoudiniPublicAPIAssetWrapper::CreateEmptyInput()
function. The function takes the input’s class as its only argument.
Command |
Function |
---|---|
|
The base class of the inputs, only use this to create subclasses for new input types |
|
A geometry input |
|
A curve input |
|
An asset input (using another instantiated asset as an input) |
|
A world input: using an actor from the world as an input |
|
A landscape as an input |
|
Fetches the existing node input of a wrapped asset |
|
Fetches the existing parameter-based input of a wrapped asset |
|
Set the node input |
|
Set the parameter-based input |
Note
The GetInputAtIndex()
and GetInputParameter()
functions return a copy of the input configuration of the wrapped asset. If you change the properties or input objects, the inputs of the wrapped asset does not automatically update. To apply the changes, the inputs have to be set again using SetInputAtIndex()
or SetInputParameter()
.
Curve Inputs ¶
Curve inputs have an additional helper class to construct a curve from points: UHoudiniPublicAPICurveInputObject
. The object has functions to set or add curve points, where each point is represented by an FTransform.
Command |
Function |
---|---|
|
Set the curve points to the specified array of FTransforms |
|
Append a point as an FTransform, to the curve |
|
Remove all points from the curve |
|
Get the curve’s points as an array of FTransforms |
You can also configure curve properties such as NURBS or Bezier and open or closed curves using UHoudiniPublicAPICurveInputObject
with the following functions:
Command |
Function |
---|---|
|
Returns true if the curve is closed |
|
Set the curve as closed |
|
Returns true if the curve is reversed |
|
Set the curve as reversed |
|
Get the curve type as an enum (Polygon, NURBs, Bezier, Points): |
|
Set the curve type using the |
|
Get the curve method as an enum (CVs, Breakpoints, Freehand): |
|
Set the curve method using an enum |
Outputs ¶
The UHoudiniPublicAPIAssetWrapper
class provides functions to access the HDA’s outputs after a cook:
Command |
Function |
---|---|
|
Returns the number of outputs for the HDA |
|
Returns the type of the output (EHoudiniOutputType: Mesh, Instancer, Landscape, Curve, Skeletal) at the given output index |
|
Populates an array of |
|
Returns the output object at the given output index identified by the |
|
Returns the output component for the object at the given output index identified by the |
|
Returns true any output that contains any current proxy mesh |
|
Returns true if the output is at the specified index |
|
Returns true if the output at the specified index and identifier is a current proxy |
Baking Outputs ¶
The UHoudiniPublicAPIAssetWrapper
class also supports baking outputs.
There are three ways approach baking:
-
Enable auto-bake which automatically bakes the output after a book and uses the bake settings configured on the instantiated HDA.
-
Manually bake all outputs on an HDA
-
Bake a specific output object only identified by its output index and identifier.
Command |
Function |
---|---|
|
Enables/disables auto-baking after a successful cook |
|
Returns true if auto-baking is enabled |
Auto-baking will automatically bake the outputs after a cook and uses the bake settings configured on the instantiated HDA.
These settings can be queried or set using the following:
Command |
Function |
---|---|
|
Sets the bake method: bake to actors, blueprints, or foliage. |
|
Gets the bake method |
|
Enable/disable automatically removing temporary HDA output from the world after a bake |
|
Return true if temporary HDA output will be removed from the world after a bake |
|
Enable/disable recentering baked output actors at their bounding box center |
|
Returns true if recentering is enabled |
|
Enable/disable bake replacement mode: if enabled the previous bake’s assets and actors are replaced (if names match) |
|
Returns true if replacement mode is enabled |
Manually bake all outputs of an HDA:
Command |
Function |
---|---|
|
Bakes all outputs with the settings configured using the wrapper |
|
Nakes all outputs but the bake settings can be passed to the function, overriding settings configured on the wrapper |
Bake a specific output object only identified by its output index and identifier.
Command |
Function |
---|---|
|
Bakes the output object specified by its output index and indentifier |
Blueprint Async Processor ¶
The instantiation and cooking of the HDAs happen asynchonously in the plugin. Delegates help notify when HDA processing enters a certain phase or when cooking is complete. This means you have to manually bind and unbind delegates while writing scripts or tools using the API. To simplify this in Blueprints, the API also includes a Blueprint Async Action node: UHoudiniPublicAPIProcessHDANode
or ProcessHDA
.
The ProcessHDA
node has all of the options of the UHoudiniPublicAPI::InstantiateAsset()
function and also provides some quality of life features:
-
Accepts a map of
FName
toFHoudiniParameterTuple
to set parameters without having to manually bind to delegates -
Accepts a map of
int32
toUHoudiniPublicAPIInput
to set index-based node iputs without having to manually bind to delegates -
Accepts a map of
FName
toUHoudiniPublicAPIInput
to set parameter-based inputs without having to manually bind to delegates -
A latent node with output pins that are bound to the various delegates such as
OnPreInstantiateDelegate
,OnPostInstantiateDelegate
, andOnPostProcessDelegate
which makes it easier to bind custom logic to these events.
A screenshot of the Process HDA node with the blueprint version of the curve input example. This is slightly modified so the MakeGeoInput
and MakeCurveInput
functions create and populate the UHoudiniPublicAPIGeoInput
and UHoudiniPublicAPICurveInput
.
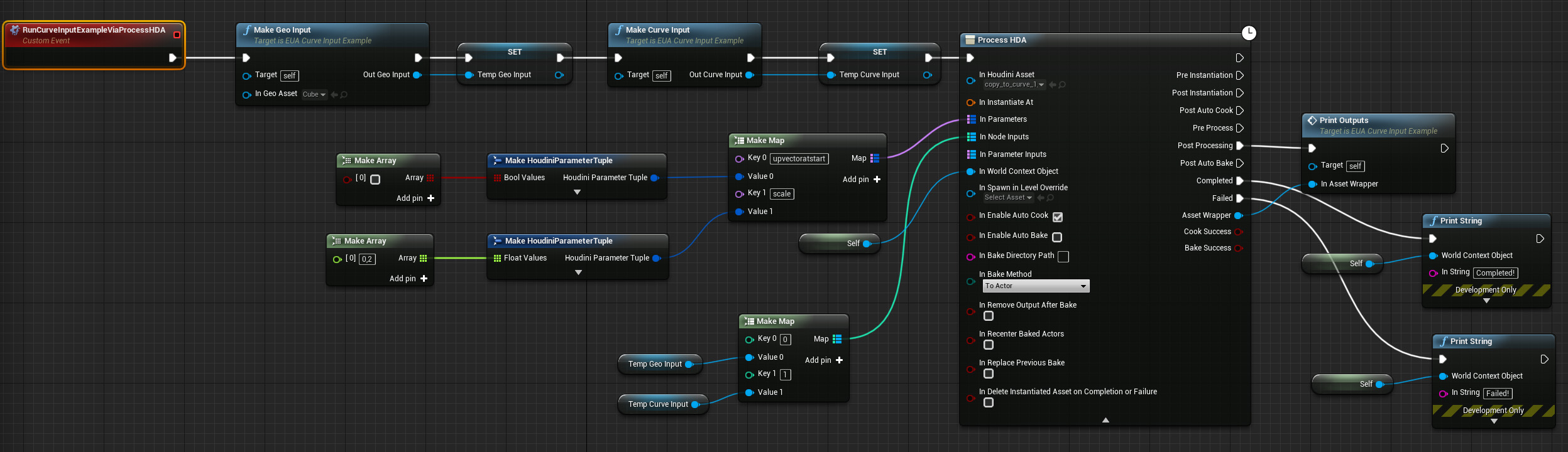
Python Async Processor ¶
Python is similar to the ProcessHDA
Blueprint node. The ProcessHDA
Python class is located in the HoudiniEngineV2.asyncprocessor
module.
The ProcessHDA
class constructor takes the same arguments as the Async Blueprint node. You start the processing by using the ProcessHDA.activate()
function.
The difference is the ProcessHDA
class has a function for each delegate:
-
on_failure
-
on_complete
-
on_pre_instantiation
-
on_post_instantiation
-
on_post_auto_cook
-
on_pre_process
-
on_post_processing
-
on_post_auto_bake
These are internally bound to a UHoudiniPublicAPIAssetWrapper
and called when the delegate broadcasts. You can subclass the ProcessHDA
class and override the above functions to implement their own functionality.
Here is our curve input example implemented as a subclass of the ProcessHDA
:
import math import unreal from HoudiniEngineV2.asyncprocessor import ProcessHDA _g_processor = None class ProcessHDAExample(ProcessHDA): def on_failure(self): print('on_failure') global _g_processor _g_processor = None def on_complete(self): print('on_complete') global _g_processor _g_processor = None def on_post_processing(self): # Print out all outputs generated by the HDA num_outputs = self.asset_wrapper.get_num_outputs() print('num_outputs: {}'.format(num_outputs)) if num_outputs > 0: for output_idx in range(num_outputs): identifiers = self.asset_wrapper.get_output_identifiers_at(output_idx) print('\toutput index: {}'.format(output_idx)) print('\toutput type: {}'.format(self.asset_wrapper.get_output_type_at(output_idx))) print('\tnum_output_objects: {}'.format(len(identifiers))) if identifiers: for identifier in identifiers: output_object = self.asset_wrapper.get_output_object_at(output_idx, identifier) output_component = self.asset_wrapper.get_output_component_at(output_idx, identifier) is_proxy = self.asset_wrapper.is_output_current_proxy_at(output_idx, identifier) print('\t\tidentifier: {}'.format(identifier)) print('\t\toutput_object: {}'.format(output_object.get_name() if output_object else 'None')) print('\t\toutput_component: {}'.format(output_component.get_name() if output_component else 'None')) print('\t\tis_proxy: {}'.format(is_proxy)) print('') def make_geo_input(): """ Makes a cube geometry input. """ # get the API singleton houdini_api = unreal.HoudiniPublicAPIBlueprintLib.get_api() # Create a geo input geo_input = houdini_api.create_empty_input(unreal.HoudiniPublicAPIGeoInput) # Set the input objects/assets for this input geo_object = unreal.load_object(None, '/Engine/BasicShapes/Cube.Cube') geo_input.set_input_objects((geo_object, )) return geo_input def make_curve_input(): """ Makes a helix input curve. """ # get the API singleton houdini_api = unreal.HoudiniPublicAPIBlueprintLib.get_api() # Create a curve input curve_input = houdini_api.create_empty_input(unreal.HoudiniPublicAPICurveInput) # Create a curve wrapper/helper curve_object = unreal.HoudiniPublicAPICurveInputObject(curve_input) # Make it a Nurbs curve curve_object.set_curve_type(unreal.HoudiniPublicAPICurveType.NURBS) # Set the points of the curve, for this example we create a helix # consisting of 100 points curve_points = [] for i in range(100): t = i / 20.0 * math.pi * 2.0 x = 100.0 * math.cos(t) y = 100.0 * math.sin(t) z = i curve_points.append(unreal.Transform([x, y, z], [0, 0, 0], [1, 1, 1])) curve_object.set_curve_points(curve_points) # Set the curve wrapper as an input object curve_input.set_input_objects((curve_object, )) return curve_input def make_parameters(): """ Make a dict of HoudiniParameterTuple containing `upvectoratstart` and `scale`. """ parameters = {} parameter_tuple = unreal.HoudiniParameterTuple() parameter_tuple.bool_values = (False, ) parameters['upvectoratstart'] = parameter_tuple parameter_tuple = unreal.HoudiniParameterTuple() parameter_tuple.float_values = (0.2, ) parameters['scale'] = parameter_tuple return parameters def run(): # Create the processor with preconfigured inputs global _g_processor _g_processor = ProcessHDAExample( unreal.load_object(None, '/HoudiniEngine/Examples/hda/copy_to_curve_1_0.copy_to_curve_1_0'), parameters=make_parameters(), node_inputs={0: make_geo_input(), 1: make_curve_input()}, ) # Activate the processor, this will start instantiation, and then cook if not _g_processor.activate(): unreal.log_warning('Activation failed.') else: unreal.log('Activated!') if __name__ == '__main__': run()
PDG/TOP Networks ¶
The Public API supports interacting with the PDG asset link if an HDA contains one or more TOP networks.
There are two delegates for PDG related events:
Command |
Function |
---|---|
|
Broadcast after cooking a TOP Network in the HDA. Work item results do not have to be loaded yet |
|
Broadcast after baking PDG outputs |
The following functions are available in UHoudiniPublicAPIAssetWrapper to interact with the PDG asset link:
Command |
Function |
---|---|
|
Returns true if the wrapped asset is valid and has a PDG asset link |
|
Gets the paths (relative to the instantiated asset) of all TOP networks in the HDA |
|
Gets the paths (relative to the specified TOP network) of all TOP nodes in the network |
|
Dirty all TOP networks in this asset |
|
Dirty the specified TOP network |
|
Dirty the specified TOP node |
|
Cook all outputs for the specified TOP network |
|
Cook the specified TOP node |
|
Bake all outputs of the instantiated asset’s PDG contexts using the settings configured on the asset |
|
Bake all outputs of the instantiated asset’s PDG contexts using the specified settings |
|
Set whether to automatically bake PDG work items after a successfully loading them |
|
Returns true if PDG auto bake is enabled |
|
Sets the bake method to use for PDG baking (to actor, blueprint, foliage) |
|
Gets the currently set bake method to use for PDG baking (to actor, blueprint, foliage) |
|
Sets outputs to bake for PDG. For example all, selected network, and selected node. |
|
Get outputs to bake for PDG. For example all, selected network, and selected node. |
|
Determines if baked actors are recentered to their bounding box center after a PDG bake on the PDG asset link. |
|
Returns true if baked actors are recentered to their bounding box center after a PDG bake on the PDG asset link. |
|
Set the replacement mode to use for PDG baking (replace previous bake output vs incrementing the output names) |
|
Get the replacement mode to use for PDG baking |
Note
PDG/TOP network cooking is not directly linked the HDAs cooking. If auto-cook is enabled for the HDA (or the asset was instantiated with bEnableAutoCook == true
), PDG will not cook automatically. The auto-cook setting for the PDG asset link is not currently exposed via the public API, so the user must call PDGCookNode()
or PDGCookOutputsForNetwork()
to cook PDG.
Additional Examples ¶
The content and examples directory of the plugin contains HDAs, Python scripts, Editor Utility Widget, and Editor Utility Actor examples.
The following C++ example is available: Command |
Function |
---|---|
|
The |
The following Blueprint examples are available:
Command |
Function |
---|---|
|
The blueprint example from Instantiate HDA using API |
|
An example editor utility widget with various options for instantiating and HDA and setting parameters |
The following Python examples are available:
Command |
Function |
---|---|
|
An example script using asset inputs |
|
An example script that bakes all outputs of the HDA after a cook |
|
An example script that bakes a specific output object to the content browser after a cook |
|
Similar exmaple to Instantiate HDA using API using an input curve |
|
The example Python script from Instantiate HDA using API |
|
An example scirpt for using geometry inputs |
|
Setting a parameter, cooking an HDA with instances as output |
|
Using landscape inputs |
|
TIterating over the outputs after cooking an HDA |
|
Cooking and baking PDG output |
|
Using the ProcessHDA class to simplify delegate handling |
|
Using the helper functions in the API for setting ramp parameter points |
|
A simple example that starts the Houdini Engine session |
|
Using world inputs |
Error Messages ¶
A common error in the public API is functions return a boolean to indicate success or failure. If an error occurred, the last error message can be retrieved with the GetLastErrorMessage()
function.
This is available on any classes that derive from UHoudiniPublicAPIObjectBase
such as UHoudiniPublicAPI
, UHoudiniPublicAPIAssetWrapper
, the input classes deriving from UHoudiniPublicAPIInput
, and UHoudiniPublicAPICurveInputObject
.