On this page |
|
Inheritance |
|
Overview ¶
A viewer is a type of pane that shows the scene. The viewer’s contents is conceptually divided into viewports. By default, the scene viewer shows a single viewport, the Perspective view. However, you can use the view layout controls to, for example, split the view into four viewports (Perspective, Top, Front, and Right views). Viewports are the parts of the interface that actually display the scene to the user.
To get a reference to a scene viewer, see the following methods:
Use hou.ui.paneTabOfType(hou.paneTabType.SceneViewer)
to get a Scene Viewer pane tab in the current pane layout. If the current layout has no Scene Viewer pane tab, this returns None
.
To be more precise about which viewer you grab in a possible multi-viewer layout, see also hou.ui.curDesktop to get a hou.Desktop object representing the current pane layout, and hou.Desktop.sceneViewers to get a list of scene viewer pane tabs in the current layout.
Methods ¶
Current selection ¶
currentGeometrySelection()
→ hou.GeometrySelection
Returns the current geometry selection if the viewer is in a geometry select mode and otherwise returns None.
setCurrentGeometrySelection(geometry_type, nodes, selections)
Replaces a current geometry selection if the viewer is in a geometry select mode and otherwise raises hou.NotAvailable. Any of the specified selections not matching geometry_type will be automatically converted to that type where possible.
currentSceneGraphSelection()
→ tuple of string
Returns a tuple of scene graph paths for primitives selection in the scene viewer.
setCurrentSceneGraphSelection(selection, fix_selection_paths=True)
Set the current scene graph primitive selection. Set fix_selection_paths
to True
to automatically fix empty or invalid paths in the selection
.
Otherwise invalid paths raise hou.ValueError.
currentGeometrySelectionParm()
→ string
Returns the current geometry selection parameter string if the viewer is
doing a scripted selection. This holds the select_parm
argument given to methods
such as selectGeometry
.
Current Highlight ¶
sceneGraphHighlight()
→ tuple of string
Returns which prims in the viewport are highlighted.
setSceneGraphHighlight(selection)
Highlight the named prims in the viewport. Passing an empty list will clear the highlight. This does not affect selection.
Prompting for a selection ¶
selectObjects(prompt='Select objects', sel_index=0, allow_drag=False, quick_select=False, use_existing_selection=True, allow_multisel=True, allowed_types=('*',), icon=None, label=None, prior_selection_paths=[], prior_selection_ids=[], prior_selections=[], toolbox_templategroup=None, toolbox1_templategroup=None, select_parm="")
→ tuple of Nodes
selectGeometry(prompt='Select geometry', sel_index=0, allow_drag=False, quick_select=False, use_existing_selection=True, initial_selection = None, initial_selection_type = None, ordered=False, geometry_types=(), primitive_types=(), allow_obj_sel=True, icon=None, label=None, prior_selection_paths=[], prior_selection_ids=[], prior_selections=[], allow_other_sops=True, consume_selections=True, toolbox_templategroup=None, toolbox1_templategroup=None, confirm_existing=False, pick_at_obj_level=False, select_parm="")
→ GeometrySelection
selectPositions(prompt='Click to specify a position', number_of_positions=1, min_number_of_positions=-1, connect_positions=True, show_coordinates=True, bbox=BoundingBox(), position_type=positionType.WorldSpace, icon=None, label=None, toolbox_templategroup=None, toolbox1_templategroup=None, select_parm="")
→ tuple of Vector3s
selectOrientedPositions(prompt='Click to specify a position', number_of_positions=1, min_number_of_positions=-1, connect_positions=True, show_coordinates=True, bbox=BoundingBox(),icon=None, label=None, toolbox_templategroup=None, toolbox1_templategroup=None, select_parm="")
→ tuple of (Vector3, Matrix3) tuples
This method is very similar to hou.SceneViewer.selectPositions, except the position type
is always world space, and instead of a tuple of positions this returns a tuple
of (position, orientation)
pairs, with the position a hou.Vector3
and the orientation a hou.Matrix3.
This allows you to prompt the user for oriented position, respecting possible orientation aids such as
the construction plane and
alignment.
selectDynamics(prompt='Select dynamics objects', sel_index=0, allow_objects=True, allow_modifiers=False, quick_select=False, use_existing_selection=True, allow_multisel=True, icon=None, label=None, prior_selection_paths=[], prior_selection_ids=[], prior_selections=[], toolbox_templategroup=None, toolbox1_templategroup=None, select_parm="")
→ tuple of hou.DopData
selectDynamicsPoints(prompt='Select dynamics points', sel_index=0, quick_select=False, use_existing_selection=True, allow_multisel=True, only_select_points=True, object_based_point_selection=False, use_last_selected_object=False, icon=None, label=None, prior_selection_paths=[], prior_selection_ids=[], prior_selections=[], toolbox_templategroup=None, toolbox1_templategroup=None, select_parm="")
→ tuple of (hou.DopData, hou.GeometrySelection)
selectDynamicsPolygons(prompt='Select dynamics polygons', sel_index=0, quick_select=False, use_existing_selection=True, object_based_point_selection=False, use_last_selected_object=False, icon=None, label=None, prior_selection_paths=[], prior_selection_ids=[], prior_selections=[], toolbox_templategroup=None, toolbox1_templategroup=None, select_parm="")
→ tuple of (hou.DopData, hou.GeometrySelection)
selectSceneGraph(prompt='Select primitives', preselection=[], prim_mask=hou.scenePrimMask.ViewerSetting, quick_select=False, use_existing_selection=True, confirm_existing=False, allow_multisel=True, allow_drag=True, propagate_selection=True, path_prefix_mask='', prim_kind='', validate_selection_for_node=None, select_parm='', allow_kind_mismatch=hou.optionalBool.NoOpinion, allow_instance_proxies=hou.optionalBool.NoOpinion, fix_preselection_paths=True)
→ tuple of string
selectSceneGraphInstances(prompt='Select point instances', preselection=[], quick_select=False, use_existing_selection=True, confirm_existing=False, allow_multisel=True, allow_drag=True, path_prefix_mask="", instance_level=0, instance_indices_only=False, validate_selection_for_node=None, select_parm="")
→ tuple of string
stageSerial()
→ int
Integer that is increased every time the Scene Graph View data is modified. Only count on this number to be bigger when changes occur, and not necessarily incremented by 1.
locateSceneGraphPrim(x, y)
→ (float, string)
Find the primitive located at at the specified pixel coordinates. Returns a tuple of screen depth at the hit location and primitive path. Returns (-1, “”) when no primitive is found.
Viewer state ¶
currentState()
→ string
Returns the name of the viewer’s current tool state.
setCurrentState(state, wait_for_exit=False, generate=hou.stateGenerateMode.Insert, request_new_on_generate=True, ex_situ_generate=False)
Sets the current tool state of the viewer. If wait_for_exit is True, then the function will not return until the viewer exits the tool.
generate
A hou.stateGenerateMode enumeration value to specify how a new node should be generated, inserted inline or into a new branch.
request_new_on_generate
Some states reuse the current node whenever possible. Setting this argument to True requests that such states generate a new node.
ex_situ_generate
Some states do things like initialize their selectors from stashed temporary selections or cook selections during generation. Such actions make sense when the state is launched in situ, but are not desired if the script first changed network levels or displayed nodes so that the viewer context the state starts with differs from the one the user sees when triggering the script. Setting this argument to True tells the state not to assume that the viewer context at the time of generation matches what the user expects.
enterCurrentNodeState(wait_for_exit=False)
Enters the viewer into the node-specific tool state of the last selected node. If wait_for_exit is True, then the function will not return until the viewer exits the tool.
enterViewState(wait_for_exit=False)
Enters the viewer into view tool state. If wait_for_exit is True, then the function will not return until the viewer exits the tool.
enterTranslateToolState(wait_for_exit=False)
Enters the viewer into translate tool state. This is available only for the node contexts that support the move tools. If wait_for_exit is True, then the function will not return until the viewer exits the tool.
enterRotateToolState(wait_for_exit=False)
Enters the viewer into rotate tool state. This is available only for the node contexts that support the move tools. If wait_for_exit is True, then the function will not return until the viewer exits the tool.
enterScaleToolState(wait_for_exit=False)
Enters the viewer into scale tool state. This is available only for the node contexts that support the move tools. If wait_for_exit is True, then the function will not return until the viewer exits the tool.
beginStateUndo(label)
Opens an undo block to perform undoable operations with the current viewer state. All operations performed after the opening will appear as one single operation on the undo stack. Use hou.SceneViewer.endStateUndo to close an open block.
beginStateUndo
and endStateUndo
can be used for managing an undo block that begins in
one function but ends in another one. Only one undo block is supported for a sequence of
undoable operations. Exceptions will be raised if a call to a second beginStateUndo
is
detected before the undo block is closed by endStateUndo
.
Python state example of a valid undo block:
def onMouseEvent(self, kwargs): ui_event = kwargs["ui_event"] node = kwargs["node"] if ui_event.reason() == hou.uiEventReason.Start: # left mouse button pressed self.scale = node.parent().parm("scale").evalAsFloat() self.scene_viewer.beginStateUndo('scale') if ui_event.reason() == hou.uiEventReason.Active: # left mouse button down while the mouse is moving self.scale *= 1.01 node.parent().parm("scale").set(self.scale) if ui_event.reason() == hou.uiEventReason.Changed: # left mouse button released self.scene_viewer.endStateUndo()
Example of a non-valid undo block that raises an exception:
def onMouseEvent(self, kwargs): ui_event = kwargs["ui_event"] node = kwargs["node"] if ui_event.reason() == hou.uiEventReason.Start: # left mouse button pressed self.tx = node.parent().parm("tx").evalAsFloat() self.scale = node.parent().parm("scale").evalAsFloat() self.scene_viewer.beginStateUndo('scale') if ui_event.reason() == hou.uiEventReason.Active: # left mouse button down while the mouse is moving self.scale *= 1.01 node.parent().parm("scale").set(self.scale) self.scene_viewer.beginStateUndo('move x') self.tx += 0.2 node.parent().parm("tx").set(self.tx) self.scene_viewer.endStateUndo() if ui_event.reason() == hou.uiEventReason.Changed: # left mouse button released self.scene_viewer.endStateUndo()
label
The label of the undo block used for displaying the undo operation in the Edit menu. An exception is raised if the label is empty.
endStateUndo()
Closes an undo block previously opened with hou.SceneViewer.beginStateUndo.
endStateUndo
will raise an exception if called before beginStateUndo
.
showHandle(name, value)
Shows or hides a display handle linked to the current tool state. This API is typically used with Python states and can be called from any python state callbacks – with one small caveat. Avoid calling showHandle
from the python state constructor, doing so will lead to a runtime error.
See also hou.Handle.show.
name
The name of the handle as specified with hou.ViewerStateTemplate.bindHandle
value
Bool
value, True
to show the handle, False
to hide it.
bindViewerHandle(handle_type, name, settings=None, cache_previous_parms=False, handle_parms=None)
Binds a dynamic viewer handle to the current viewer state similarly to hou.ViewerStateTemplate.bindHandle. Unlike bindHandle
though, this method creates and
initializes the handle in a dynamic fashion, there is no need to register in advance the handle with the viewer state. You can call bindViewerHandle
from almost anywhere in Houdini (from the viewer state handlers, from a python script, etc…), however it can only be called while the viewer state is running.
To remove the viewer handles added with bindViewerHandle
, use hou.SceneViewer.unbindViewerHandle. Houdini will take care of removing automatically all viewer
handles added with bindViewerHandle
when the state exits, there is no need do it by yourself.
This method works only with python states, an exception is raised if the state is not a python state.
handle_type
A string naming the type of handle. See State handle types for a list of handle types to choose from.
name
A string to use to identify the handle. Each binding’s name must be unique within this state. Trying to bind the same name more than once will raise an exception.
settings
A string containing specific settings of the handle. Multiple settings must be space separated.
cache_previous_parms
If True, the handle retains a record of previous values. This can be useful if you want your code to calculate deltas as the user moves the handle, for example to know how fast the user is dragging the handle.
handle_parms
An array of handle parm names to specify the parms to enable, only the parms contained in the array will be available to the python state callbacks.
All handle parms are enabled when handle_parms
is empty (default).
bindViewerHandleStatic(handle_type, name, bindings, settings=None)
Binds a static viewer handle to the current viewer state similarly to hou.ViewerStateTemplate.bindHandleStatic. Unlike bindHandleStatic
though, this method creates and
initializes the handle in a dynamic fashion, there is no need to register in advance the handle with the viewer state. You can call bindViewerHandleStatic
from almost anywhere in Houdini (from the viewer state handlers, from a python script, etc…), however it can only be called while the viewer state is running.
To remove the viewer handles added with bindViewerHandleStatic
, use hou.SceneViewer.unbindViewerHandle. Houdini will take care of removing automatically all viewer
handles added with bindViewerHandleStatic
when the state exits, there is no need do it by yourself.
This method works only with python states, an exception is raised if the state is not a python state.
handle_type
A string naming the type of handle.
name
A unique string to use to identify the handle. Each binding’s name must be unique within this state. Trying to bind the same name more than once will raise an exception.
bindings
A list of ("node_parm_name", "handle_parm_name")
tuples. This binds the parts of the handle to individual parameters on the node.
settings
A string containing specific settings of a handle. Multiple settings must be space separated.
unbindViewerHandle(name)
Removes a viewer handle from the current python state. unbindViewerHandle
can only unbind the viewer handles that were dynamically added with hou.SceneViewer.bindViewerHandle or
hou.SceneViewer.bindViewerHandleStatic. An exception is raised if the viewer handle to unbind is a state registered viewer handle.
This method works only with python states, an exception is raised if the state is not a python state.
name
The viewer handle name to unbind. This is the name used when binding the handle with bindViewerHandle
or bindViewerHandleStatic
.
openVisualizerEditor(visualizer)
If the provided ViewportVisualizer exists, open the interactive visualizer editor dialog for that visualizer.
openOptionDialog(path, label)
Opens Display Options dialog and highlight the specified label.
path
A colon-separated string that specifies the tab and group(s) the label belongs to. E.g, “Geometry:Volume Quality”.
label
The label to be highlighted.
triggerStateSelector(action, name=None)
Triggers an action on a selector of the current state. The state must be a Python state type or an exception is thrown.
action
The type of action to trigger as specified with hou.triggerSelectorAction.
name
The name of a selector to trigger, should match the name used for registering the selector, see hou.ViewerStateTemplate.bindGeometrySelector or hou.ViewerStateTemplate.bindObjectSelector for details.
The value can be empty (default) in which case the state first registered selector is triggered. An exception is thrown if name
is not a known selector.
currentStateSelector()
→ string
Returns the name of the current state selector. If no selector is active, an empty string is returned. The state must be a Python state type or an exception is thrown.
runStateCommand(name, args=None)
Executes a command implemented by the active python state. A state command can be invoked by specifying a name identifier along with input arguments.
An exception is thrown if the state is not a Python state type or the state is not running.
name
The command name identifier.
args
A python
object holding the command specific arguments. Defaults to None.
An implementation example of a state command.
import hou import viewerstate.utils as su class State(object): def __init__(self, state_name, scene_viewer): self.state_name = state_name self.scene_viewer = scene_viewer self.text_size = 5 self.text_color = 'yellow' # drawable for drawing a text string in the viewport self.text_drawable = hou.TextDrawable(self.scene_viewer, 'text_drawable_name', params = {'margins': (10.0,-10.0), 'origin' : hou.drawableTextOrigin.UpperLeft, 'multi_line' : True} ) self.text_drawable.show(True) def onCommand( self, kwargs ): # Command to update the viewport text name = kwargs['command'] args = kwargs['command_args'] state_parms = kwargs['state_parms'] if name == 'update_text': # update settings state_parms['text']['value'] = args['text']; self.text_color = args['color'] self.text_size = args['size'] # force a viewport redraw self.scene_viewer.curViewport().draw() def onDraw( self, kwargs ): # Draw the text in the viewport upper left handle = kwargs['draw_handle'] state_parms = kwargs['state_parms'] (x,y,width,height) = self.scene_viewer.curViewport().size() text = '<font color="%s", size=%d>%s</font>' % (self.text_color, self.text_size, state_parms['text']['value']) self.text_drawable.draw( handle, params = {'text': text, 'translate': (0.0, height, 0.0)} ) def registerState(): state_typename = kwargs['type'].definition().sections()['DefaultState'].contents() state_label = "State command demo" state_cat = hou.objNodeTypeCategory() template = hou.ViewerStateTemplate(state_typename, state_label, state_cat) template.bindFactory(State) template.bindParameter( hou.parmTemplateType.String, name="text", label="Text", default_value="Lorem ipsum dolor sit amet." ) hou.ui.registerViewerState(template) def unregisterState(): state_typename = kwargs['type'].definition().sections()['DefaultState'].contents() try: hou.ui.unregisterViewerState(state_typename) except hou.StateNotRegistered: pass except: raise
Here’s how to invoke the command.
import toolutils args = { 'text': text, 'color': color, 'size': size } toolutils.sceneViewer().runStateCommand( 'update_text', args = args )
geometryVisibility(sop_node)
Return False
if setGeometryVisibility was called to hide the geometry or True
otherwise.
Raises hou.InvalidInput if the geometry isn’t in the current viewport.
setGeometryVisibility(sop_node, on)
Control a viewport visibility override for a given geometry node.
This can be used by a Python State to temporary hide the viewport geometry
when a state draws an alternate visualization using drawables.
The viewport geometry visibility doesn’t persist and is reverted to True
when switching the display flag or reselecting nodes.
Raises hou.InvalidInput if the geometry isn’t in the current viewport.
selectDrawableGeometry(drawable_selection, selection_modifier=hou.pickModifier.Replace)
Performs a drawable geometry selection. This method works only when a drawable selector is running, it
can be called either from the python state implementing the selector or from a regular python script. Calling this method
will trigger the python state’s onSelection
handler.
Raises hou.OperationFailed if no drawable selector is running or hou.InvalidInput if the input arguments are invalid.
drawable_selection
A dictionary describing the drawable elements to select, the content of the dictionary is described here.
selection_modifier
The selection modifier for the selection. Defaults to hou.pickModifier.Replace.
Modeling ¶
constructionPlane()
→ hou.ConstructionPlane
Return the construction plane (or grid) in the perspective viewport of this viewer.
See hou.ConstructionPlane for more information.
referencePlane()
→ hou.ReferencePlane
Return the reference plane (or grid) in the perspective viewport of this viewer.
See hou.ReferencePlane for more information.
showCurrentSopGeometry(value)
Set the geometry display flag, on or off, on the current SOP node. When the flag is on, the viewport displays the geometry attached to the node, or hides when it’s off. The flag is on by default when a SOP state enters.
Consider this scenario, the display flag is set to true on a downstream node, but an upstream
node is selected. When you enter the node’s state, normally the viewport will show the upstream
node’s geometry in wireframe, but the display node in shaded. In some cases, the state wants to
set parameters on the selected node whose result is best visualized in the downstream node. In
this case, showCurrentSopGeometry
can be used to turn off the display of the upstream node’s
geometry.
Note
For SOP Python states, Houdini remembers the current node’s geometry display flag when the state enters and will set it back to its original value when the state exits.
isShowingCurrentSopGeometry(value)
→ bool
Returns the current node’s geometry display flag. See hou.SceneViewer.showCurrentSopGeometry.
Split views ¶
curViewport()
→ hou.GeometryViewport
Returns this viewer’s current viewport. The current viewport is the one containing the mouse cursor. If the cursor is not in a viewport, then the selected, or active, viewport is returned.
selectedViewport()
→ hou.GeometryViewport
Returns this viewer’s selected viewport. Viewports can be selected by holding Space and pressing the N key.
viewportLayout()
→ hou.geometryViewportLayout
Returns the current viewport layout as a hou.geometryViewportLayout value.
setViewportLayout(layout, single=-1)
Sets the viewer’s viewport layout.
layout
A hou.geometryViewportLayout value. For example, to set the viewer to show four viewports at the corners:
viewer_pane.setViewportLayout(hou.geometryViewportLayout.Quad)
single
If you specify the /hom/hou/hou.geometryViewportLayout.html#Single layout, this argument lets you specify which of the four viewports from the quad view to show as the single
|
Use the current viewport (the viewport the mouse is/was over). |
|
Use the top-left viewport from the quad layout (usually the Top view). |
|
Use the top-right viewport from the quad layout (usually the Perspective view). |
|
Use the bottom-left viewport from the quad layout (usually the Front view). |
|
Use the bottom-right viewport from the quad layout (usually the Right view). |
setPromptMessage(msg, msg_type=promptMessageType.Prompt)
Sets the viewport to display a message at the bottom of the screen.
msg
Text message to display.
msg_type
A hou.promptMessageType value representing the type of message to display. Defaults to hou.promptMessageType.Prompt.
clearPromptMessage()
Clear the prompt message previously set with hou.SceneViewer.setPromptMessage.
flashMessage(imagefile, msg, duration=1.0, [Hom:hou.GeometryViewport] = None)
Flashes a message in the upper left corner of the scene viewer. The image file specified will be scaled and placed before the message. It will fade out over the specified duration in seconds. An optional hou.GeometryViewport can be used to draw in a specific viewport of the viewer.
hudInfo(show=-1, state=None, template=None, values=None, panel=hou.hudPanel.ToolInfo, terminate=False, update_hotkey_bindings=False)
Creates, updates, or controls a read-only heads-up-display panel in the viewer. The HUD panel extends across viewport divisions.
This interface is experimental and subject to change. See Python state info panels for more information.
-
Houdini will automatically clear the HUD panel when the tool/state changes.
-
Houdini will automatically hide/show the panel when the user presses ⇧ Shift + F1.
show
Show the HUD if True or hide it if False. This option is a tri-state option and is ignored by default.
state
Specifies the display state for the hou.hudPanel.ToolInfo panel. See hou.hudInfoState for details.
template
A dictionary describing the HUD template to format the information to display.
values
A dictionary of values for updating the information displayed by the HUD.
panel
Identifier for specifying the layout and home location of the HUD. Defaults to hou.hudPanel.ToolInfo.
terminate
Delete the HUD identified with panel
and remove it from the viewport if currently displayed, other arguments are ignored. Defaults to False.
update_hotkey_bindings
Will redo the lookup of keys bound to hotkey symbols when True.
See viewer HUD info panels for more information.
hotkeyAssignments(hotkey_symbols)
→ tuple
of tuple
of str
Return a tuple of strings that represent the hotkeys currently assigned to
each action associated with a tuple of hotkey symbols. The key strings are
of the form returned by the hou.ui.hotkeys method, which is a
combination of the symbol on the key, and any modifier keys involved, such
as "Ctrl+Shift+G"
.
Snapping ¶
snappingMode()
setSnappingMode(snapping_mode)
isSnappingToCurrentGeometry()
Returns whether snapping to current geometry is enabled for the current snapping mode.
setSnapToCurrentGeometry(on)
Sets whether or not snapping to current geometry is enabled for the current snapping mode.
isSnappingToTemplates()
setSnapToTemplates(on)
isSnappingToOtherObjects()
setSnapToOtherObjects(on)
isSnappingToGuides()
→ bool
Returns whether snapping to guides is enabled for the current snapping mode.
setSnapToGuides(on)
Sets whether or not snapping to guides is enabled for the current snapping mode.
isDepthSnapping()
setDepthSnapping(on)
isOrientingOnSnap()
setOrientOnSnap(on)
snappingGravity()
: → float
Returns the gravity value for the current snapping mode.
snappingPriorities()
: → tuple of hou.snappingPriority values
Returns a tuple of hou.snappingPriority values for the current snapping mode. The values are sorted in order of priority (descending) as set in the Snap options window. Returns an empty tuple if the mode has no priorities.
View options ¶
isPickingVisibleGeometry()
Returns true if the viewer is configured to only pick visible components when performing an area-based selection. This option corresponds to the matching check box in the component selection button’s context menu.
setPickingVisibleGeometry(on)
Turns on or off the option to select only visible components when performing area-based selections such as box or brush picking.
isPickingContainedGeometry()
Returns true if the viewer is configured to only pick fully contained components when performing an area-based selection. This option corresponds to the matching check box in the component selection button’s context menu.
setPickingContainedGeometry(on)
Turns on or off the option to select only fully contained components when performing area-based selections such as box or brush picking.
selectionMode()
Returns the selection mode of the viewer of type hou.selectionMode.
setSelectionMode(selection_mode)
Sets the selection mode of this view. The value for 'selection_mode' must be from hou.selectionMode.
isGroupPicking()
Returns true if group, attribute, or connectivity information will be used to automatically expand selections made in this viewer. This option corresponds to the matching check box in the component selection button’s context menu.
setGroupPicking(on)
Turns on or off the group, attribute, or connectivity based picking. When turned on, the group list gadget is automatically made visible.
isWholeGeometryPicking()
Returns true if selections made in this viewer will automatically expand to include the whole geometry. This option corresponds to the matching check box in the component selection button’s context menu.
setWholeGeometryPicking(on)
Turns on or off the option to expand selections made in this viewer to include the entire geometry.
isSecureSelection()
Returns true if secure selection is turned on in this viewer. This option corresponds to the secure selection option in the select tool’s context menu.
setSecureSelection(on)
Turns on or off the secure selection option in this viewer.
isPickingCurrentNode()
Returns true if selections made in this viewer will pick from the Current SOP. If not, the pick occurs on the Display SOP. This option corresponds to the current/display option in the component selection button’s context menu.
setPickingCurrentNode(on)
Tells this viewer whether picks should be made on the Current SOP or on the Display SOP.
pickGeometryType()
Returns the type of geometry that will be picked in this viewer. This option corresponds to the component types selectable in the component selection button’s context menu.
setPickGeometryType(geometry_type)
Sets the type of geometry that will be picked in this viewer. This value can be changed at any time by the user, or when a selector is invoked.
pickStyle()
Returns the style of area picking currently being used by this viewer. This option corresponds to the picking style specified in the select tool’s context menu.
setPickStyle(style)
Sets the style of area picking to be used by this viewer.
pickModifier()
Returns the manner in which additional selection are combined with the existing selection. Consider using hou.SceneViewer.toolPickModifier instead if you want the pick modifer as selected in the select tool menu.
setPickModifier(modifier)
Sets the method used to modify the existing selection when a new selection
is made. Modifier keys can still be used to alter this behavior. Only the
default operation (with no modifier keys) is affected by this setting.
Note that the select state will revert to the default pick modifier upon
termination, so to make your change persist beyond this you will also need
to change this default using setDefaultPickModifier()
.
defaultPickModifier()
Returns the default pick modifier to which Houdini will revert after a select state terminates.
setDefaultPickModifier(modifier)
Sets the default pick modifier to which Houdini will revert after a select state terminates.
pickFacing()
Returns a value indicating whether the user is able to pick front facing components, back facing components, or both. This option corresponds to the front and back facing options in the component selection button’s context menu.
setPickFacing(facing)
Sets the option of whether to restrict selection to front facing, back facing, or either type of components.
activePickModifier()
This method is similar to hou.SceneViewer.pickModifier but returns the pick modifier as selected in the select tool menu.
isCreateInContext() -> bool
isWorldSpaceLocal() -> bool
Returns true if values that would normally be in world space are actually
in the local space of the current object. This includes things like the
return values of selectPositions()
, hou.GeometryViewport::mapToScreen()
,
hou.GeometryViewport::mapToWorld(),
hou.GeometryViewport().viewPivot()
,
etc.
LOP Viewer Options ¶
isViewingSceneGraph()
→ bool
Returns True
if the scene viewer is viewing a USD stage in LOPs.
stage()
→ Usd.Stage
Returns the USD stage being displayed, if the scene viewer is viewing LOPs.
setShowGeometry(show)
Show or hide the scene geometry when viewing LOPs.
showGeometry()
→ bool
Query if the scene geometry is displayed when viewing LOPs.
setShowCameras(show)
Show or hide the camera guides when viewing LOPs.
showCameras()
→ bool
Query if the camera guides are displayed when viewing LOPs.
setShowLights(show)
Show or hide the light guides when viewing LOPs.
showLights()
→ bool
Query if the light guides are displayed when viewing LOPs.
setShowSelection(show)
Show the selection highlight when viewing LOPs.
showSelection()
→ bool
Query if the selection highlight is displayed when viewing LOPs.
showRenderStats()
→ bool
Query if the renderer statistics are shown when viewing LOPs. Not all renderers may provide this information.
showRenderStats(show)
Show or hide the renderer statistics are shown when viewing LOPs.
showRenderTime()
→ bool
Query if the render time and progress is shown when viewing LOPs. Not all renderers may provide this information.
showRenderStats(show)
Query if the renderer time and progress are shown when viewing LOPs.
setSelectionKind(kind)
Set the kind of primitive selection to be performed. 'kind' can be one of the USD primitive kinds (component, subcomponent, model, group, assembly, leaf primitive) or a custom kind. An exception is thrown if kind is not recognized. Case sensitivity is ignored. This will set the selection mode to primitive selection if point instance selection was active.
selectionKind()
→ str
Query the current primitive kind that would be selected if the user entered the select state. This will return a null string if leaf primitives would be selected.
setSelectionAllowKindMismatch(allow)
Allow selection operations to return primitives that do not match the current selectionKind
. When set to False
, primitives returned from a selection will always be of the requested kind, even if this means that a user selection returns no results. For example, if the selectionKind
is assembly
, and the user click on a mesh that is part of a component
model, but that component
is not inside an assembly
, nothing will be selected. When set to True
, the selectionKind
is treated as a hint rather than a requirement. In this mode, the user’s selection will always return something, even if that means violating the requested selectionKind
. In the previously given example, the selection operation would return the group
primitive closest to the root of the hierarchy. Or if the component
is not in a group
, the component
prim would be returned. Or if the user clicked on a primitive that is not part of a USD model hierarchy at all (such as a Camera primitive), the leaf primitive would be returned.
selectionAllowKindMismatch()
Query the current state of the flag indicating whether selection operations can return primitives that do not match the current selectionKind
.
setSelectionAllowInstanceProxies(allow)
Allow selection operations to return instance proxy primitives. If set to True
, instance proxy primitives are selected and returned from a selection operation exactly like any other primitive. If False
, then before applying the selectionKind
requirement each leaf primitive in a selection operation is traversed upwards through the hierarchy until a non-instance proxy primitive is found. Then the selectionKind
requirement is enforced starting from this instance root primitive.
Many LOP operations are not allowed on instance proxy primitives (since opinions expressed on such primitives are ignored by the USD composition system). So it is often useful to prevent the user from selecting such unsupported primitives in the first place.
selectionAllowInstanceProxies()
Query the state of the flag indicating if selections may return instance proxy primitives.
setSelectionAllowHiddenPrims(allow)
Allow selection operations to return hidden primitives. If set to True
, hidden primitives can be selected and returned from a selection operation. If False
, then before applying the selectionKind
requirement each leaf primitive in a selection operation is traversed upwards through the hierarchy until a non-hidden primitive is found. Then the selectionKind
requirement is enforced.
A hidden primitive is one that has been marked with special metadata in the scene graph tree. Hidden primitives are not normally displayed in the scene graph tree, and so it is usually desirable to also prevent them from being directly selected in the viewer. The hidden flag is often used specifically to control primitive selection to ensure that transforms and other edits occur only at desirable locations in the scene graph hierarchy, acoording to the conventions of your pipeline.
selectionAllowHiddenPrims()
Query the state of the flag indicating if selections may return hidden primitives.
setSelectionPointInstances(topmost)
Switches to point instance selection mode. If 'topmost' is 'True', the topmost point instance will be selected when selecting nested instances. Otherwise the bottommost point instance (leaf instance) will be selected. If point instances are not nested, 'topmost' has no effect.
isSelectingPointInstances()
→ bool
Return True
if the current selection state is selecting point instances, or False
if it is selecting primitives.
isSelectingTopmostInstance()
→ bool
Query if the instance selection mode is selecting the topmost instance level (True
) or the leaf instance level (False
).
hydraRenderers()
→ tuple of str
Query the list of renderers that are available to LOPs.
setHydraRenderer(ren_name)
Set the current renderer in LOPs.
currentHydraRenderer()
→ str
Query the current renderer in LOPs.
isRendererPaused()
→ bool
Return True
if the active LOP render delegate has been paused.
setRendererPaused(paused)
Pauses or resumes the active LOPs render delegate. This is equivalent to
choosing the Pause Render
or Resume Render
menu items in the viewport’s
renderer menu.
restartRenderer()
Restart the current renderer in LOPs, which causes the scene to be rebuilt from scratch.
resetViewportCamera()
Reset the native viewport camera to a default state. This clears any camera settings inherited from the most recently chosen USD camera.
This method is equivalent to selecting Reset Native Viewport Camera
in the viewport’s camera menu.
showProxyPurpose(show_proxy, renderer = None)
Show (True
) or hide prims tagged with the Proxy purpose in LOPs.
This value is stored separately for each renderer. If renderer
is
None
, the value for the currently selected renderer is set.
Otherwise renderer is a string indicating the name of the renderer.
showGuidePurpose(show_guide, renderer = None)
Show (True
) or hide prims tagged with the Guide purpose in LOPs.
This value is stored separately for each renderer. If renderer
is
None
, the value for the currently selected renderer is set.
Otherwise renderer is a string indicating the name of the renderer.
showRenderPurpose(show_render, renderer = None)
Show (True
) or hide prims tagged with the Render purpose in LOPs.
This value is stored separately for each renderer. If renderer
is
None
, the value for the currently selected renderer is set.
Otherwise renderer is a string indicating the name of the renderer.
useViewportOverrides(use_overrides, renderer = None)
Control whether the viewer should apply overrides (such as draw mode and visibility) set in the scene graph tree.
This value is stored separately for each renderer. If renderer
is
None
, the value for the currently selected renderer is set.
Otherwise renderer is a string indicating the name of the renderer.
useViewportLoadMasks(use_loadmasks, renderer = None)
Control whether the viewer should apply load masks (such as payload loading) set in the scene graph tree.
This value is stored separately for each renderer. If renderer
is
None
, the value for the currently selected renderer is set.
Otherwise renderer is a string indicating the name of the renderer.
usePostLayers(use_postlayers, renderer = None)
Control whether the viewer should apply post-layers from the LOP Network.
This value is stored separately for each renderer. If renderer
is
None
, the value for the currently selected renderer is set.
Otherwise renderer is a string indicating the name of the renderer.
showingProxyPurpose(renderer = None)
→ bool
Return True
if prims with the Proxy purpose are being shown in LOPs.
This value is stored separately for each renderer. If renderer
is
None
, the value for the currently selected renderer is returned.
Otherwise renderer is a string indicating the name of the renderer.
showingGuidePurpose(renderer = None)
→ bool
Return True
if prims with the Guide purpose are being shown in LOPs.
This value is stored separately for each renderer. If renderer
is
None
, the value for the currently selected renderer is returned.
Otherwise renderer is a string indicating the name of the renderer.
showingRenderPurpose(renderer = None)
→ bool
Return True
if prims with the Render purpose are being shown in LOPs.
This value is stored separately for each renderer. If renderer
is
None
, the value for the currently selected renderer is returned.
Otherwise renderer is a string indicating the name of the renderer.
usingViewportOverrides(renderer = None)
→ bool
Return True
if the viewer is applying overrides (such as draw mode
and visibility) set in the scene graph tree.
This value is stored separately for each renderer. If renderer
is
None
, the value for the currently selected renderer is returned.
Otherwise renderer is a string indicating the name of the renderer.
usingViewportLoadMasks(renderer = None)
→ bool
Return True
if the viewer is applying load masks (such as payload
loading) set in the scene graph tree.
This value is stored separately for each renderer. If renderer
is
None
, the value for the currently selected renderer is returned.
Otherwise renderer is a string indicating the name of the renderer.
usingPostLayers(renderer = None)
→ bool
Return True
if the viewer is applying post-layers set on the LOP Network.
This value is stored separately for each renderer. If renderer
is
None
, the value for the currently selected renderer is returned.
Otherwise renderer is a string indicating the name of the renderer.
stageControlsPlaybar()
→ bool
Return True
if the metadata from the stage shown in this viewer is used
to control the playbar range and playback rate. Otherwise return False
.
setStageControlsPlaybar(controls_playbar)
Specify whether the metadata from the stage shown in this viewer should be used to set the playbar range and playback rate. Only one viewer may have this option enabled at a time. Turning it on for one viewer will turn it off for all other viewers.
If enabled, the stage’s StartTimeCode
, EndTimeCode
, and
TimeCodesPerSecond
values are used to set the start frame, and frame, and
FPS options of the playbar respectively. The playbar step option is set to
a value of TimeCodesPerSecond
/FramesPerSecond
from the stage metadata.
The Houdini playbar more closely corresponds to the USD concept of “timecodes” than “frames”. This makes it much easier to author animated USD data.
sceneGraphStageLocked()
→ bool
Return True
if the viewer’s USD stage is locked to its current state, so
that it does not update when changes are made to the LOP Network. This is
similar to the Manual Update Mode, but only affects this viewer pane. All
other Houdini panes, such as the Scene Graph Tree or Scene Graph Details
will continue to update with new stage contents from the LOP network.
setSceneGraphStageLocked(locked)
Set the flag indicating that this viewer should not update its stage contents in response to changes in the LOP Network.
Group list ¶
isGroupListVisible()
Returns true if the group list gadget has been turned on for this viewer. This function only refers to the option to show the group list when not selecting groups in the viewer. In that case the group list will be visible, but this function may still return False.
setGroupListVisible(on)
Turns on or off the group list gadget for this viewer.
isGroupListColoringGeometry()
Returns true if the group list gadget is configured to color geometry in the viewer based on group membership or attribute value.
setGroupListColoringGeometry(on)
Turns on or off the group list gadget to coloring of geometry in the viewer based on group membership or attribute value.
isGroupListShowingEmptyGroups()
Returns true if the group list gadget is including empty groups in its list.
setGroupListShowingEmptyGroups(on)
Turns on or off the display of empty groups in the group list gadget.
isGroupListShowingOnlyPreSelectedGroups()
Returns true if the group list is showing only groups that contain one or more selected or pre-selected components.
setGroupListShowingOnlyPreSelectedGroups(on)
Turns on or off the trimming of the group list to show only groups that contain one or more selected or pre-selected components.
isGroupListCondensingPathHierarchies()
Returns true if the group list will condense the hierarchy of groups defined by a string attribute representing a path.
setGroupListCondensingPathHierarchies(on)
Turns on or off the condensing of the hierarchy in the group list. This applies to components grouped by string attributes representing a path. Turning this option on can fit more information into the list, but can make it harder to distinguish levels in the hierarchy.
groupListSize()
Returns the width and height in inches of the group list gadget.
setGroupListSize(width, height)
Sets the size in inches of the group list gadget.
groupListType()
Returns the type of component listed in the group list gadget.
setGroupListType(group_list_type)
Sets the type of component listed in the group list gadget. This can be set to a specific component type or to follow the current component selection type.
groupListMask()
Returns true if the group list gadget has been turned on for this viewer.
setGroupListMask(mask)
Sets the mask value in the group list gadget for this viewer. This can be a filter applied to the component groups, or an attribute name (starting with an @ character), or a connectivity type.
Memories and snapshots ¶
Quick renders and flipbooks ¶
flipbookSettings()
→ hou.FlipbookSettings
Access to the flipbook dialog settings, which can be queried, set, or copied.
flipbook(viewport=None, settings=None, open_dialog=False)
Capture a flipbook. A flipbook creates a quick preview animation by taking consecutive screenshots of a viewport at each frame.
Launch a flipbook for viewport
if specified or the current viewport if
viewport
is not. Optionally a hou.FlipbookSettings object can be passed to
settings
which will override the current settings. This override will
only affect the current flipbook and not change the dialog settings.
If open_dialog
is True, the flipbook dialog is presented to the user,
otherwise a flipbook is launched immediately.
Window ¶
qtWindow()
→ QtWidgets.QWidget
Create and return a new Qt Window for the scene viewer.
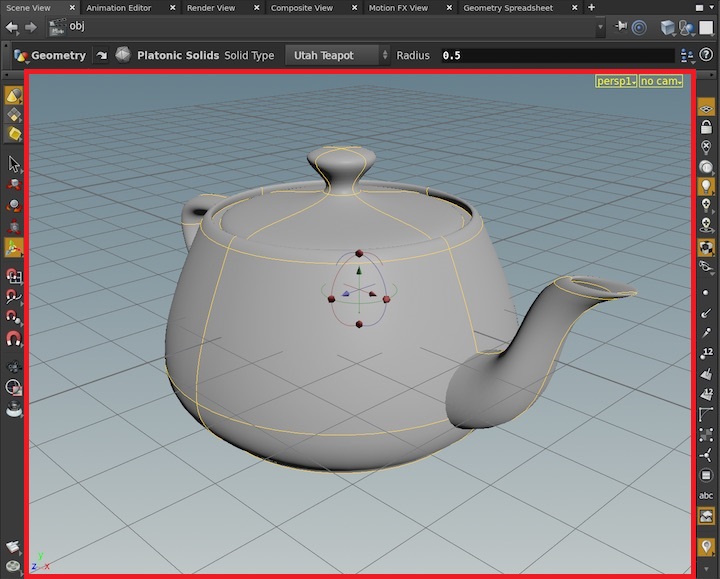
The returned window is parented to the main Qt window and can be used for parenting a Qt widget like a dialog or a window.
geometry()
→ tuple of
int
Returns the position and size of the viewer in the UI space. The tuple elements are returned in viewport coordinates, relative to the lower-left corner position of the main window.
-
X
position (lower left) -
Y
position (lower left) -
Width
dimension -
Height
dimension
Color Correction ¶
These functions set or query the OpenColorIO settings for the viewer (see OpenColorIO Support).
usingOCIO()
→ bool
Query if OpenColorIO is being used for color correction in the viewer.
setUsingOCIO(enable)
Enable or disable OpenColorIO for color correction in the viewer.
setOCIODisplayView(display="", view="")
Set the OpenColorIO display name, view name, or both. The display and view together define the output colorspace for the viewer, and any number of color transforms (Looks) to be performed on the linear viewport image.
getOCIODisplay()
→ str
Return the current OpenColorIO display used for color correction.
getOCIOView()
→ str
Return the current OpenColorIO view used for color correction.
Toolbars ¶
showOperationBar(self,show)
Show or hide the Operation Toolbar at the top of the viewport.
isShowingOperationBar()
→ bool
Return True if the Operation Toolbar is shown at the top of the viewport.
showColorCorrectionBar(self,show)
Show or hide the Color Correct Toolbar at the bottom of the viewport.
isShowingColorCorrectionBar()
→ bool
Return True if the Color Correction Toolbar is shown at the bottom of the viewport.
showMemoryBar(self,show)
Show or hide the Memory Toolbar at the bottom of the viewport.
isShowingMemoryBar()
→ bool
Return True if the Memory Toolbar is shown at the bottom of the viewport.
showDisplayOptionsBar(self,show)
Show or hide the Display Options Toolbar to the right side of the viewport.
isShowingDisplayOptionsBar()
→ bool
Return True if the Display Options Toolbar is shown to the right side of the viewport.
showSelectionBar(self,show)
Show or hide the Selection Toolbar to the left side of the viewport.
isShowingSelectionBar()
→ bool
Return True if the Selection Toolbar is shown to the left side of the viewport.
setIncludeColorCorrectionBar(self,on)
Include the optional Color Correction Toolbar at the bottom of the viewport.
includeColorCorrectionBar()
→ bool
Return True if optional Color Correction Toolbar is included at the bottom of the viewport.
setIncludeMemoryBar(self,on)
Include the optional Memory Toolbar at the bottom of the viewport.
includeMemoryBar()
→ bool
Return True if optional Memory Toolbar is included at the bottom of the viewport.
Interaction ¶
runShelfTool(tool_name)
Run the named shelf tool in the current viewport.
displayRadialMenu(name)
Launch the named radial menu in the current viewport.
Metadata ¶
viewerType()
→ hou.stateViewerType
enum value
Returns the type of the viewer, either Scene
for a viewer looking at Objects, SOPs, or DOPs; or SceneGraph
for a viewer looking at LOPs.
fullName()
→ str
Returns the full name of the viewer formatted as desktop.name.category
# Full name of a viewer in the technical desktop looking at Objects, SOPs or DOPs Technical.panetab2.world # Full name of a viewer in the technical desktop looking at LOPs Technical.panetab2.solaris
Callbacks ¶
addEventCallback(callback)
Register a Python callback to be called whenever viewer and geometry viewport events occur.
callback
Any callable Python object that expects keyworded arguments specific to an event type. A single callback can listen to all viewer events.
The callback is called with a dictionary containing these items:
-
event_type: The viewer event.
-
desktop: The desktop object holding the scene viewer.
-
viewer: The scene viewer object listening to this event.
Extra items are available for specific events:
Item name |
Description |
Events |
---|---|---|
|
The selected viewport object |
|
|
The state name |
|
|
The state name which is interrupting the current state or resuming the interrupted state. |
|
|
Preference name |
|
|
The registry name containing the preference. |
|
def onViewerCB(**kwargs): event_type=kwargs['event_type'] desktop=kwargs['desktop'] viewer=kwargs['viewer'] print( "event type=",event_type ) print( "desktop=",desktop ) print( "viewer=",viewer ) curSceneViewer = [item for item in hou.ui.curDesktop().currentPaneTabs() if item.type() == hou.paneTabType.SceneViewer][0] curSceneViewer.addEventCallback(onViewerCB)
clearEventCallbacks()
Remove all Python callbacks that have been registered with hou.sceneViewerEvent.addEventCallback.
removeEventCallback(self,callback)
Remove a specific Python callback that have been registered with hou.sceneViewerEvent.addEventCallback.
eventCallbacks()
→ tuple
of callbacks
Return a tuple of all the Python callbacks that have been registered with hou.sceneViewerEvent.addEventCallback.
Methods from hou.PaneTab ¶
name()
→ str
Return the name of this tab.
setName(name)
Set the name of this pane tab. A pane tab name may contain spaces.
Note that this name is the internal name of the tab, and is different from the label displayed in the interface.
type()
→ hou.paneTabType enum value
Return the type of this tab (i.e. whether it is a scene viewer, parameter editor, network editor, etc.).
setType(type)
→ hou.PaneTab
Create a new pane tab of the given type, replace this tab with it, and return the new pane tab. Use the returned pane tab afterward; references to this tab become invalid.
close()
Close the pane tab.
pane()
→ hou.Pane or None
Return the pane in the desktop that contains this pane tab. Note that pane tabs in regular floating panels are always in a pane, since regular floating panels contain one or more panes.
However, some floating panels have their content stripped down to only contain one particular pane tab type, and do not display the user interface to add more pane tabs, split the pane, etc. This method returns None for these stripped down floating panels.
floatingPanel()
→ hou.FloatingPanel or None
Return the floating panel that contains this pane tab or None if the pane tab is not in a floating panel.
isCurrentTab()
→ bool
Return whether this tab is the selected tab in the containing pane.
setIsCurrentTab()
Set this tab as the selected tab in the containing pane.
isFloating()
→ bool
Return whether this pane tab is in a floating panel.
This method can be approximately implemented as follows:
def isFloating(self): return self.pane() is None or self.pane().floatingPanel() is not None
clone()
→ hou.PaneTab
Create a floating copy of the pane tab and return the cloned pane tab. The new pane tab is in a new floating panel.
linkGroup()
→ hou.paneLinkType enum value
Return the link group that this pane tab belongs to.
See also hou.PaneTab.isPin.
setLinkGroup(group)
Set the link group membership of this pane tab.
isPin()
→ bool
Return whether this pane tab is pinned. This method is equivalent to
(self.linkGroup() == hou.paneLinkType.Pinned)
See also hou.PaneTab.linkGroup.
setPin(pin)
If pin is True
, set the link group membership to hou.paneLinkType.Pinned.
Otherwise, set it to hou.paneLinkType.FollowSelection. This method can be
implemented using hou.PaneTab.setLinkGroup as follows:
def setPin(self, pin): if pin: self.setLinkGroup(hou.paneLinkType.Pinned) else: self.setLinkGroup(hou.paneLinkType.FollowSelection)
See also hou.PaneTab.setLinkGroup.
size()
→ tuple
of int
Return a 2-tuple containing the pane tab’s width and height.
The width and height include the content area, network navigation control area (if any) and borders.
The width and height do not include the pane tab’s tab area.
contentSize()
→ tuple
of int
Return a 2-tuple containing the pane tab’s content area width and height.
The width and height do not include the network navigation control area (if any), pane tab borders or tab area.
hasNetworkControls()
→ bool
Return True if this pane tab type supports network controls.
isShowingNetworkControls()
→ bool
Return whether this pane tab is showing its network control bar. Return False if the pane tab doesn’t have network controls. See also hou.PaneTab.hasNetworkControls.
setShowNetworkControls(pin)
Show or Hide the network control bar. Has no effect if the pane tab doesn’t have network controls. See also hou.PaneTab.hasNetworkControls.
This method is deprecated in favor of showNetworkControls
.
showNetworkControls(pin)
Show or Hide the network control bar. Has no effect if the pane tab doesn’t have network controls. See also hou.PaneTab.hasNetworkControls.
qtParentWindow()
→ QWidget
Return a PySide2.QtWidgets.QWidget
instance that represents the window
containing the pane tab.
qtScreenGeometry()
→ QRect
Return the geometry of the pane as a PySide2.QtCore.QRect
object. The x
and y
properties of the returned QRect
object
point to the top-left corner of the pane in screen coordinates.
Methods from hou.PathBasedPaneTab ¶
cd(path)
currentNode()
→ Node
pwd()
→ Node
setCurrentNode(node, pick_node = True)
setPwd(node)